Functions in c
A function is a block of code that performs a particular task. There are times when we need to write a particular block of code for more than once in our program. This may lead to bugs and irritation for the programmer. C language provides an approach in which you need to declare and define a group of statements once and that can be called and used whenever required. This saves both time and space.
C functions can be classified into two categories,
- Library functions
- User-defined functions
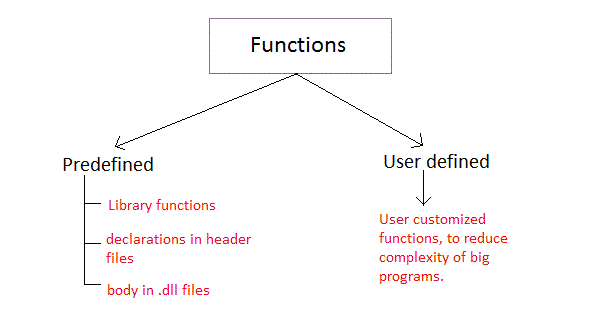
Library functions are those functions which are defined by C library, example printf(), scanf(), strcat() etc. You just need to include appropriate header files to use these functions. These are already declared and defined in C libraries.
User-defined functions are those functions which are defined by the user at the time of writing program. Functions are made for code reusability and for saving time and space.
EXAMPLES::
C Standard Library Functions
C Standard library functions or simply C Library functions are inbuilt functions in C programming. Function prototype and data definitions of these functions are written in their respective header file. For example: If you want to use
printf()
function, the header file <stdio.h>
should be included.#include <stdio.h>
int main()
{
/* If you write printf() statement without including header file, this program will show error. */
printf("Catch me if you can.");
}
There is at least one function in any C program, i.e., the
main()
function (which is also a library function). This program is called at program starts.
There are many library functions available in C programming to help the programmer to write a good efficient program.
Suppose, you want to find the square root of a number. You can write your own piece of code to find square root but, this process is time consuming and the code you have written may not be the most efficient process to find square root. But, in C programming you can find the square root by just using
sqrt()
function which is defined under header file "math.h"
Use Of Library Function To Find Square root
#include <stdio.h>
#include <math.h>
int main(){
float num,root;
printf("Enter a number to find square root.");
scanf("%f",&num);
root=sqrt(num); /* Computes the square root of num and stores in root. */
printf("Square root of %.2f=%.2f",num,root);
return 0;
}
Example of user-defined function
Write a C program to add two integers. Make a function
add
to add integers and display sum inmain()
function./*Program to demonstrate the working of user defined function*/
#include <stdio.h>
int add(int a, int b); //function prototype(declaration)
int main(){
int num1,num2,sum;
printf("Enters two number to add\n");
scanf("%d %d",&num1,&num2);
sum=add(num1,num2); //function call
printf("sum=%d",sum);
return 0;
}
int add(int a,int b) //function declarator
{
/* Start of function definition. */
int add;
add=a+b;
return add; //return statement of function
/* End of function definition. */
}
Function prototype(declaration):
Every function in C programming should be declared before they are used. These type of declaration are also called function prototype. Function prototype gives compiler information about function name, type of arguments to be passed and return type.
Syntax of function prototype
return_type function_name(type(1) argument(1),....,type(n) argument(n));
In the above example,
int add(int a, int b);
is a function prototype which provides following information to the compiler:- name of the function is
add()
- return type of the function is
int
. - two arguments of type
int
are passed to function.
Function prototype are not needed if user-definition function is written before
main()
function.Function call
Control of the program cannot be transferred to user-defined function unless it is called invoked.
Syntax of function call
function_name(argument(1),....argument(n));
In the above example, function call is made using statement
add(num1,num2);
from main()
. This make the control of program jump from that statement to function definition and executes the codes inside that function.Function definition
Function definition contains programming codes to perform specific task.
Syntax of function definition
return_type function_name(type(1) argument(1),..,type(n) argument(n)) { //body of function }
Function definition has two major components:
1. Function declarator
Function declarator is the first line of function definition. When a function is called, control of the program is transferred to function declarator.
Syntax of function declarator
return_type function_name(type(1) argument(1),....,type(n) argument(n))
Syntax of function declaration and declarator are almost same except, there is no semicolon at the end of declarator and function declarator is followed by function body.
In above example,
int add(int a,int b)
in line 12 is a function declarator.2. Function body
Function declarator is followed by body of function inside braces.
Passing arguments to functions
In programming, argument(parameter) refers to data this is passed to function(function definition) while calling function.
In above example two variable, num1 and num2 are passed to function during function call and these arguments are accepted by arguments a and b in function definition.

Arguments that are passed in function call and arguments that are accepted in function definition should have same data type. For example:
If argument num1 was of int type and num2 was of float type then, argument variable a should be of type int and b should be of type float,i.e., type of argument during function call and function definition should be same.
A function can be called with or without an argument.
Return Statement
Return statement is used for returning a value from function definition to calling function.
Syntax of return statement
return (expression);
For example:
return a; return (a+b);
In above example, value of variable add in
add()
function is returned and that value is stored in variable sum in main()
function. The data type of expression in return statement should also match the return type of function.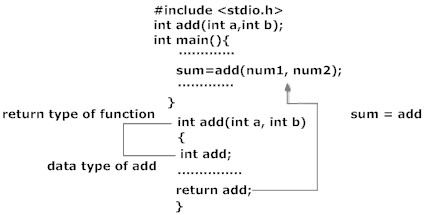
No comments:
Post a Comment